[텐서플로우로 시작하는 딥러닝 기초] Lab 04: Multi-variable Linear Regression 를 TensorFlow 로 구현하기
2020. 9. 1. 21:05ㆍ머신러닝
아래의 데이타를 사용하여 다중선형회귀를 텐서플로우로 구현해보자.
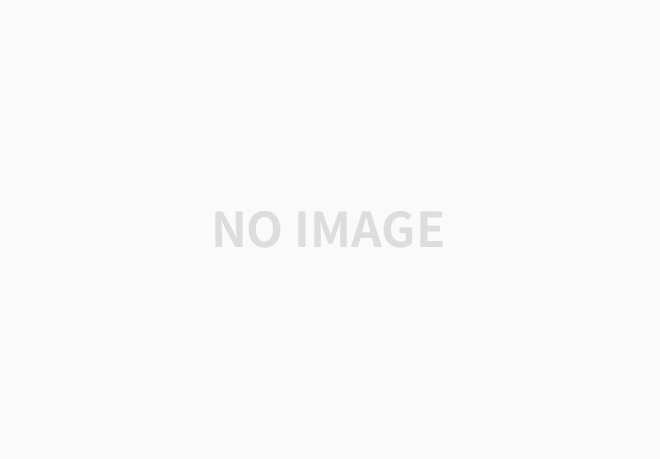
import tensorflow as tf
import numpy
# data and Label
x1 = [73., 93., 89., 96., 73.]
x2 = [80., 88., 91., 98., 66.]
x3 = [75., 93., 90., 100., 79.]
y = [152., 185., 180., 196., 142.]
# weights
w1 = tf.Variable(tf.random.normal([1])) # [1]은 shape. 1 크기의 난수
w2 = tf.Variable(tf.random.normal([1]))
w3 = tf.Variable(tf.random.normal([1]))
b = tf.Variable(tf.random.normal([1]))
learning_rate = 0.000001
for i in range(1001):
#tf.GradientTape() 는 선언된 변수로 구성된 식을 해당 변수로 자동 미분하는 기능을 제공한다.
with tf.GradientTape() as tape:
hypothesis = w1 * x1 + w2 * x2 + w3 * x3 + b
cost = tf.reduce_mean(tf.square(hypothesis - y))
#해당 weight로 cost을 미분한 값(기울기)들을 저장
w1_grad, w2_grad, w3_grad, b_grad = tape.gradient(cost, [w1, w2, w3, b])
#update w1,w2,w3 and b
w1.assign_sub(learning_rate * w1_grad)
w2.assign_sub(learning_rate * w2_grad)
w3.assign_sub(learning_rate * w3_grad)
b.assign_sub(learning_rate * b_grad)
if i % 50 == 0:
print("{:4} | {:12.4f}".format(i, cost.numpy()))
출력은 아래와 같다.
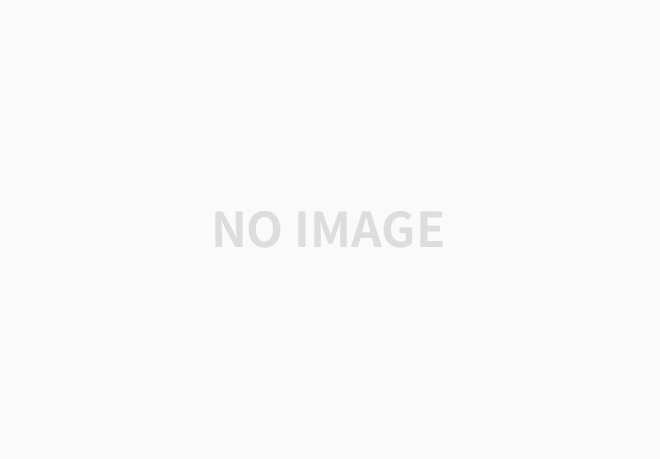
위의 코드를 Matrix를 이용하여 더 간단하게 바꿔보자.
import tensorflow as tf
import numpy as np
data = np.array([
# X1, X2, X3, y
[73., 80., 75., 152. ],
[93., 88., 93., 185. ],
[89., 91., 90., 180. ],
[96., 98., 100., 196. ],
[73., 66., 70., 142. ]
], dtype=np.float32)
# slice data
X = data[:, :-1] #data에서 row는 전체, column은 전체의 -1까지 를 X로 잘라가겠다.
y = data[:, [-1]] #data에서 row는 전체, column은 끝에서 1열을 y로 잘라가겠다.
W = tf.Variable(tf.random.normal([3, 1])) #weight는 3*1의 난수행렬
b = tf.Variable(tf.random.normal([1])) #bias는 상수
def hypothesis(X):
return tf.matmul(X, W) + b
n_epochs = 2000 #epoch(학습의 한 회차)의 수
learning_rate = 0.000001
for i in range (n_epochs+1):
with tf.GradientTape as tape:
cost = tf.reduce_mean(tf.square(hypothesis(X) - y))
W_grad, b_grad = tape.gradient(cost, [W, b])
W.assign_sub(learning_rate * W_grad)
b.assign_sub(learning_rate * b_grad)
if i % 100 == 0:
print("{:4} | {:12.4f}".format(i, cost.numpy()))
인데.... 사용하는 구름IDE에서 지원하는 CPU의 성능을 초과하는 지, 실행오류를 일으킨다. 이런 문제라면 앞으로도 사지방에서 공부하기는 쉽지 않을 것같다. 하모니카OS라는 리눅스 기반 OS인데 권한을 막아놔서 쉘도 실행시키지 못하는 문제가 있어서 Win10 PC가 사용 가능해질 때 까지는 클라우드 IDE를 사용해야 한다. 코드에 대한 이해는 되었으니 일단 넘어가자.
확실히 Matrix를 이용한 코드가 간결하다.